INTRODUCTION TO NODEPATHS
A nodepath is a mathematical entity that allows you to
distinguish between multiple occurrences of the same node due to
instancing. This allows you to indicate a specific occurrence of
a node in the scene graph. There are two things you can do with
nodepaths:
- Perform intersection tests between specific nodepaths and
other nodes in the scene graph
- Pick graphical entities rendered into the WTK window. The
WTK picking functions generate the nodepath of a picked
geometry node.
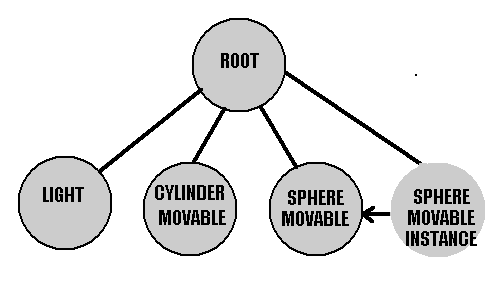
figure 19
In figure 19 two nodepaths can be generated so that when
picking you can pick the spheres.
Creating Nodepaths
WTnodepath_new
Use this function to create a new nodepath. A nodepath is
fully specified by giving the bottom-most node in interest,
the ancestor node, and the occurrence number.
SYNTAX:
WTnodepath_new(WTnode *node, WTnode *ancestor, int
which);
ARGUMENTS:
node -- bottom-most node
ancestor -- The ancestor
which -- The occurrence of the node.
RETURN TYPE:
Returns a pointer to a new WTnodepath
Picking
WTK provides functions to allow the user to pick a
geometry in the render window using a pointing device such as
the mouse. The WTwindow_pickpoly function allows the user to
specify a 2d screen coordinate and will pick the front-most
polygon the appears beneath that point.. You can obtain the
2d screen point by using the mouse raw data structure and
miscellaneous data structure.
Getting the Mouse Data
All sensors have miscellaneous and raw data structures
that information returned by the sensor. In the case of the
mouse, the miscellaneous data structure contains information
about which button has been pressed on the mouse, and the raw
data structure will contain the 2d screen coordinate of where
the mouse is currently in the window. Use the functions
WTsensor_getrawdata and WTsensor_getmiscdata to obtain this
information
WTsensor_getmiscdata
Use this function to obtain the miscellaneous
information associated with a sensor. In the case of the
mouse button press information is contained in the
miscellaneous data structure.
SYNTAX:
int WTsensor_getmiscdata(WTsensor *sensor);
ARGUMENTS:
sensor -- A pointer to a type WTsensor to get
the misc. data from.
RETURN TYPE:
integer containing the miscellaneous data. In the case
of the mouse it will be one of the predefined constants
WTMOUSE_LEFTBUTTON
WTMOUSE_LEFTDOWN
WTMOUSE_LEFTUP
WTMOUSE_RIGHTBUTTON
WTMOUSE_RIGHTDOWN
WTMOUSE_RIGHTUP
WTMOUS_MIDDLEBUTTON
WTMOUSE_MIDDLEDOWN
WTMOUSE_MIDDLEUP
EXAMPLE: -- Getting mouse clicks. This example
assumes that you have a sensor object called mouse that
has been initialized with the WTmouse_new function.
..
if(WTsensor_getmiscdata(mouse) &
WTMOUSE_LEFTBUTTON)
WTmessage("Left button pressed");
..
Notice the we use a bitwise and operator (&) to
test for the comparison.
WTsensor_getrawdata
Use this function to obtain the rawdata structure
associated with a sensor. For the mouse the current 2d screen
coordinate will be contained in the rawdata structure.
SYNTAX:
void *WTsensor_getrawdata(WTsensor *sensor);
ARGUMENTS:
sensor -- A pointer to the sensor in question
RETURN TYPE:
The sensor specific raw data structure. The return
needs to be typecast appropriately before the contents of
the structure can be accessed.
EXAMPLE -- Getting 2D mouse points. This example assumes
that you have a sensor object called mouse that has been
initialized with the WTmouse_new function.
.
WTmouse_rawdata *rawdata;
if(WTsensor_getmiscdata(mouse) &
WTMOUSE_LEFTBUTTON)
rawdata = (WTmouse_rawdata)WTsenosr_getrawdata(mouse);
.
Assignment 17
project definition:
- Creates a universe with a bordered window in monoscopic
mode..
- Create a cylinder that has a height of 40, a radius of 5,
12 tessellation lines, is single sided and gouraud
shaded.
- Create a sphere with a radius of 10, 12 lat and long
divisions, is single sided, and gouraud shaded.
- Create a movable geometry node for the cylinder whose
parent is the root node.
- Create a movable geometry nodes for the sphere whose
parent is the root node.
- Create and instance of the movable node containing the
sphere as the 2nd child of the root node
- Create a global flag that will be set TRUE when in
pickmode.
- Create a mouse handler function that is called from
the action function when pickmode the FLYMODE flag is set
FALSE.
- The mouse_handler function should find out if the
left or right mouse button was pressed and obtain the 2d
screen coordinate and print it to the console window
- Move all the keyboard interaction code into its own
function called handle_key. This function should only be
called from the action function of a key were pressed on
the keyboard. As an argument is should receive the value
of the key pressed and return TRUE if the key was acted
upon otherwise is should return FALSE.
- Set the translation of the movable geometry containing
the first sphere to (0,-20,0)
- Set the translation of the movable geometry containing
the second sphere to (0,20,0)
- Set the rgb color of the sphere to red (255,0,0)
- Set the rgb color of the cylinder to blue (0,0,255)
- Set the rendering style of the sphere geometry to
wireframe.
- Attach to two spheres to the cylinder
- Prebuild all the geometries
- Zooms the view in the window
- Create a task function that is associated with the node
containing the cylinder. The function should rotate the
cylinder in its parent reference frame around the Y axis
by 15 degrees each time through the simulation loop.
- Add the mouse to the universe as a sensor
- Attach the mouse to the viewpoint using a motionlink
- Read a file called lights into the universe. Make sure
the file is located in the current working directory.
- Load the material table name wtmatlib.mat. (make sure you
include the wtmatlib.h header file into your program)
This header file contains define statement that relate a
color name to an index number in the wtmatlib.mat file.
- Have all geometries reference that material table file
- Set the material table index for the cylinder to PURPLE
- Set the material table index for the spheres to RED
- Create an action function that allows the user to:
- press q to quit
- press p to print the scene graph from the root
node. (use WTnode print)
- press i to obtain the extents, midpoint, and
radius of each node
- press I to obtain the extents, midpoint, and
radius of each geometry.
- press t to translate the spheres and cylinder
along their local X axis.
- Create a function that uses the WTnode_translate
function and takes as an argument the node to
translate, three floating point values for the
translation values, and the frame in which to
translate.
- Press f on the keyboard to toggle the motionlink
from enabled to disabled and disabled to enabled.
You should also print a message to the user
indicating weather they are in pick or navigation
mode.
- This should also toggle the flymode flag.
- Press a on the keyboard to decrease the default
ambient light value in the universe
- Press A on the keyboard to increase the default
ambient light value in the universe
- Move all the keyboard interaction into its own
function called handle_key.
- The user should be informed of the current
ambient light level in the universe.
Picking graphical objects
WTK allows the user to get the polygon that is the front-most
polygon under a 2ds screen point.
WTwindow_pickpoly
Use this function to get a pointer to a WTpoly that is the
front-most polygon under the 2d coordinate passed in.
SYNTAX:
WTpoly *WTwindow_pickpoly(WTwindow *win, WTp2
screenpoint, WTnodepath *nodepath, WTp3 point3d);
ARGUMENTS:
window -- A pointer to a type WTwindow that the
poly is in
screenpoint -- A WTp2 containing the 2d screen
coordinate
nodepath -- A pointer to a nodepath that will receive
the information concerning what node was picked. You can
pass NULL to this argument and WTK will not figure out
the nodepath for you.
Point3d -- A 3d point in world coordinates at which
the selected poly was intersected.
RETURN TYPE:
A pointer to the WTpoly that was picked
Getting the graphical object from the
nodepath
WTnodepath_getnode
Use this function to access the nodes of a nodepath.
SYNTAX:
WTnode *WTnodepath_getnode(int num);
ARGUMENTS:
num -- the number of the node in the nodepath.
To get the bottommost node pass in WTnodepath_numnodes()
- 1;
RETURN TYPE
A pointer to a type WTnode containing the node
information.
EXAMPLE:
.
WTmouse_raw *rawdata;
WTnodepath *nodepath[1];
WTp3 point3d;
WTnode *pickednode;
if(WTsensor_getmiscdata(mouse) & WTMOUSE_LEFTBUTTON)
rawdata = (WTmouse_rawdata)WTsenosr_getrawdata(mouse);
WTwindow_pickpoly(WTuniverse_getcurrwindow(), rawdata,
nodepath, point3d);
pickednode = WTnodepath_getnode(nodepath[0],
WTnodepath_numnodes(nodepath[0]) - 1);
WTnode_boundingbox(pickednode, TRUE);
.
Assignment 18
project definition:
- Creates a universe with a bordered window in monoscopic
mode..
- Create a cylinder that has a height of 40, a radius of 5,
12 tessellation lines, is single sided and gouraud
shaded.
- Create a sphere with a radius of 10, 12 lat and long
divisions, is single sided, and gouraud shaded.
- Create a second sphere just like the one created
above.
- Create a movable geometry node for the cylinder whose
parent is the root node.
- Create a movable geometry node for the sphere whose
parent is NULL.
- Change your instance of a the sphere to a regular
node whose parent is NULL
- Use WTmovnode_attach to attach the two spheres to
the cylinder
- Create a new global flag called SPINFLAG that is
initially set to FALSE
- Create a global flag that will be set TRUE
when in flymode.
- Create a mouse handler function that is called from
the action function when pickmode the FLYMODE flag is set
FALSE.
- Modify the mouse_handler function to return a
pointer to a type WTpoly.
- Add functionality to the mouse handler that will
pick a polygon with the right mouse button and a node
with the left mouse button. If a node is picked turn on
the bounding box for that node. if a polygon is picked
set its material table index to WHITE. Use the
WTpoly_setmatid function and WTpoly_getmatid functions.
If a new node is picked the old selected nodes bounding
box should be shut off and if a new poly is picked its
material table index should be set back to the original
index number it had before it was picked. You SHOULD NOT
be able to have both a node and a polygon highlighted at
the same time.
- Make sure that your mouse handler returns a pointer
to the polygon obtained with WTwindow_pickpoly
- Move all the keyboard interaction code into its own
function called handle_key. This function
should only be called from the action function
of a key were pressed on the keyboard. As an argument is
should receive the value of the key pressed and return
TRUE if the key was acted upon otherwise is should return
FALSE.
- Set the translation of the movable geometry containing
the first sphere to (0,-20,0)
- Set the translation of the movable geometry containing
the second sphere to (0,20,0)
- Set the rgb color of the sphere to red (255,0,0)
- Set the rgb color of the cylinder to blue (0,0,255)
- Set the rendering style of the sphere geometry to
wireframe.
- Attach to two spheres to the cylinder
- Prebuild all the geometries
- Zooms the view in the window
- Create a task function that is associated with the node
containing the cylinder. The function should rotate the
cylinder in its parent reference frame around the Y axis
by 15 degrees each time through the simulation loop.
- Add the mouse to the universe as a sensor
- Attach the mouse to the viewpoint using a motionlink
- Read a file called lights into the universe. Make sure
the file is located in the current working directory.
- Load the material table name wtmatlib.mat. (make sure you
include the wtmatlib.h header file into your program)
This header file contains define statement that relate a
color name to an index number in the wtmatlib.mat file.
- Have all geometries reference that material table file
- Set the material table index for the cylinder to PURPLE
- Set the material table index for the spheres to RED
- Create an action function that allows the user to:
- press q to quit
- press p to print the scene graph from the root
node. (use WTnode print)
- press i to obtain the extents, midpoint, and
radius of each node
- press I to obtain the extents, midpoint, and
radius of each geometry.
- press t to translate the spheres and cylinder
along their local X axis.
- Create a function that uses the WTnode_translate
function and takes as an argument the node to
translate, three floating point values for the
translation values, and the frame in which to
translate.
- Press f on the keyboard to toggle the motionlink
from enabled to disabled and disabled to enabled.
You should also print a message to the user
indicating weather they are in pick or navigation
mode.
- This should also toggle the flymode flag.
- Press s on the keyboard to toggle the value
of SPINFLAG
- Press a on the keyboard to decrease the default
ambient light value in the universe
- Press A on the keyboard to increase the default
ambient light value in the universe
- Move all the keyboard interaction into its own
function called handle_key.
- The user should be informed of the current
ambient light level in the universe.
- In the action function if SPINFLAG is TRUE
rotate the cylinder around its local Y axis by PI
divided by 4 (PID4)